Log Into Vault and Display Information Example (C#)
This example shows how to create an application that displays a form where a
user can select a vault in which to log in and display
information about that vault.
NOTE: If using the .NET Framework 4.0 primary interop assembly
provided with SOLIDWORKS Enterprise PDM, see
Using .NET Framework 4.0 in
Stand-alone Applications.
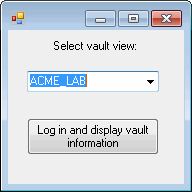
//----------------------------------------------------------------------------
// Preconditions:
// 1. Start Microsoft Visual Studio 2010.
// a. Click File > New > Project > C# > Windows Forms Application.
// b. Type Exercise1CSharp in Name.
// c. Click Browse and browse to the folder where to create the project.
// d. Click OK.
// e. Click Show All Files in the Solution Explorer toolbar and expand
// Form1.cs in the Solution Explorer.
// f. Replace the code in Form1.cs with this code.
// g. To create the form, replace the code in Form1.Designer.cs with this code.
// 2. Add EPDM.Interop.epdm.dll as a reference (right-click the project
// name in the Solution Explorer, select Add Reference, select
// Framework in the left-side panel, browse to the top folder of your
// SOLIDWORKS Enterprise PDM installation, locate and select
// EPDM.Interop.epdm.dll, click Open, and click Add).
// 3. Add EPDM.Interop.EPDMResultCode.dll as a reference (click Browse, locate and
// select EPDM.Interop.EPDMResultCode.dll, click Open, and click Add).
// 4. Add Microsoft.VisualBasic as a reference (click
// COM in the left-side panel, click
// Microsoft.VisualBasic, click Add, and click Close).
// 5. Right-click EPDM.Interop.epdm in References, select Properties, and set
// Embed Interop Types to False to handle methods that pass arrays of
// structures.
// 6. Repeat step 5 for EPDM.Interop.EPDMResultCode.
// 7. Click Debug > Start Debugging or press F5.
//
// Postconditions:
// 1. Displays a dialog.
// 2. Select a vault.
// 3. Click Log in and display vault information.
// A message box pops up containing the version of
// SOLIDWORKS Enterprise PDM installed on your computer.
// 4. Click OK to close the message box.
// Another message box pops up containing the path of the selected
// vault view folder.
// 5. Click OK to close the message box.
// 6. Type the name of a nonexistent vault in the drop-down combo box.
// 7. Click Log in and display vault information.
// 8. A message box pops up instructing you to type or select
// a valid database.
// 9. Click OK to close the message box.
// 10. Close the dialog box.
//----------------------------------------------------------------------------
//Form1.cs
using System;
using System.Windows.Forms;
using System.Diagnostics;
using System.Runtime.InteropServices;
using EPDM.Interop.epdm;
using EPDM.Interop.EPDMResultCode;
using Microsoft.VisualBasic;
namespace Exercise1CSharp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
void Exercise1CSharp_Load(System.Object sender, System.EventArgs e)
{
try
{
//Declare and create an instance of IEdmVault5
IEdmVault5 vault1 = new EdmVault5();
//Cast IEdmVault5 to IEdmVault8
IEdmVault8 vault = (IEdmVault8)vault1;
EdmViewInfo[] Views = null;
vault.GetVaultViews(out Views, false);
VaultsComboBox.Items.Clear();
foreach (EdmViewInfo View in Views)
{
VaultsComboBox.Items.Add(View.mbsVaultName);
}
if (VaultsComboBox.Items.Count > 0)
{
VaultsComboBox.Text = (string)VaultsComboBox.Items[0];
}
}
catch (System.Runtime.InteropServices.COMException ex)
{
MessageBox.Show("HRESULT = 0x" + ex.ErrorCode.ToString("X") + " " + ex.Message);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void LoginButton_Click(System.Object sender, System.EventArgs e)
{
try
{
//Declare and create an instance of IEdmVault5 object
IEdmVault5 vault = new EdmVault5();
//Log into the selected vault as the current user
vault.LoginAuto(VaultsComboBox.Text, this.Handle.ToInt32());
//Display the SOLIDWORKS Enterprise PDM version
int VerMajor = 0;
int VerMinor = 0;
vault.GetVersion(ref VerMajor, ref VerMinor);
MessageBox.Show("SOLIDWORKS Enterprise PDM " + "version is " + VerMajor.ToString() + "." + VerMinor.ToString());
//Display the path of the vault view folder
MessageBox.Show("The path of the vault view " + "folder is \"" + vault.RootFolderPath + "\".");
}
catch (System.Runtime.InteropServices.COMException ex)
{
switch (ex.ErrorCode)
{
case (int)EdmResultErrorCodes_e.E_EDM_CANT_OPEN_DATABASE:
MessageBox.Show("Could not open database. Select or type the name of valid database.");
break;
default:
MessageBox.Show("HRESULT = 0x" + ex.ErrorCode.ToString("X") + " " + ex.Message);
break;
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}
//Form1.Designer.cs
namespace Exercise1CSharp
{
partial class Form1
{
/// <summary>
/// Required designer variable
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used
/// </summary>
/// <param name="disposing">True if managed resources should be disposed; otherwise false</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor
/// </summary>
private void InitializeComponent()
{
this.VaultsLabel = new System.Windows.Forms.Label();
this.VaultsComboBox = new System.Windows.Forms.ComboBox();
this.LoginButton = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// VaultsLabel
//
this.VaultsLabel.AutoSize = true;
this.VaultsLabel.Location = new System.Drawing.Point(62, 23);
this.VaultsLabel.Name = "VaultsLabel";
this.VaultsLabel.Size = new System.Drawing.Size(75, 13);
this.VaultsLabel.TabIndex = 1;
this.VaultsLabel.Text = "Select a vault:";
//
// VaultsComboBox
//
this.VaultsComboBox.FormattingEnabled = true;
this.VaultsComboBox.Location = new System.Drawing.Point(22, 54);
this.VaultsComboBox.Name = "VaultsComboBox";
this.VaultsComboBox.Size = new System.Drawing.Size(184, 21);
this.VaultsComboBox.TabIndex = 2;
//
// LoginButton
//
this.LoginButton.Location = new System.Drawing.Point(37, 100);
this.LoginButton.Name = "LoginButton";
this.LoginButton.Size = new System.Drawing.Size(146, 37);
this.LoginButton.TabIndex = 4;
this.LoginButton.Text = "Log in and display vault information";
this.LoginButton.UseVisualStyleBackColor = true;
this.LoginButton.Click += new System.EventHandler(this.LoginButton_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(227, 184);
this.Controls.Add(this.LoginButton);
this.Controls.Add(this.VaultsComboBox);
this.Controls.Add(this.VaultsLabel);
this.Name = "Form1";
this.Load += new System.EventHandler(this.Exercise1CSharp_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label VaultsLabel;
private System.Windows.Forms.ComboBox VaultsComboBox;
private System.Windows.Forms.Button LoginButton;
}
}