-
Start
Microsoft Visual Studio 2010.
-
Click File > New >
Project > Visual C# > Class Library.
-
Select .NET Framework 2.0 in
the dropdown at the top of the dialog.
-
Type a project name in Name.
-
Click Browse and navigate to the folder where to
create the project.
-
Click OK.
-
Right-click the project name in the Solution Explorer
and click Add Reference.
-
Click COM in the
left-side panel, click PDMWorks Enterprise nnnn Type Library, and click
Add.
-
Click Assemblies > Framework in the left-side
panel, click Microsoft.VisualBasic,
and
click Add.
-
Click Close.
-
Right-click the project name in the Solution Explorer
and click Properties.
-
Click Application > Assembly Information.
-
Click
Make assembly COM-Visible.
-
Click
OK.
-
Double-click Class1.cs in the Solution Explorer
to open the code window.
-
At the top of the code window, type:
using EdmLib;
using Microsoft.VisualBasic;
-
Replace:
public class Class1
with:
public class Class1 : IEdmAddIn5
-
In the code, right-click
IEdmAddIn5 and click Implement Interface > Implement Interface.
-
Replace the add-in's
implementation of
IEdmAddIn5::GetAddInInfo with the following code:
public void GetAddInInfo(ref EdmAddInInfo poInfo, IEdmVault5 poVault, IEdmCmdMgr5 poCmdMgr)
{
//Specify
information to
display in the add-in's Properties dialog box
poInfo.mbsAddInName = "Menu command sample";
poInfo.mbsCompany = "SOLIDWORKS Corporation";
poInfo.mbsDescription = "Adds menu command items";
poInfo.mlAddInVersion = 1;
//Specify the
minimum required version of SOLIDWORKS Enterprise PDM
poInfo.mlRequiredVersionMajor = 5;
poInfo.mlRequiredVersionMinor = 2;
//Register
menu commands; SOLIDWORKS Enterprise PDM passes command IDs, 1000 and 1001,
//to IEdmAddIn5::OnCmd to indicate which command the
user selects
poCmdMgr.AddCmd(1000, "First command", (int)EdmMenuFlags.EdmMenu_Nothing, "This is the first command", "First command", 0, 99);
poCmdMgr.AddCmd(1001, "Second command", (int)EdmMenuFlags.EdmMenu_MustHaveSelection, "This is the second command", "Second command", 1, 99);
}
The flag
EdmMenuFlags.EdmMenu_MustHaveSelection
means that
the second command is only available if the user has selected one or more
files or folders.
-
IEdmAddIn5::OnCmd
is called when a menu command is selected by the user.
Replace the add-in's
implementation of
IEdmAddIn5::OnCmd with the following code:
public void OnCmd(ref EdmCmd poCmd, ref Array ppoData)
{
//Handle
the menu command
{
string CommandName = null;
if (poCmd.mlCmdID == 1000)
{
CommandName = "The first command.";
}
else
{
CommandName = "The second command.";
}
//Retrieve the bounds of the array containing the selected files and folders
int index = 0;
int last = 0;
index = Information.LBound(ppoData);
last = Information.UBound(ppoData);
string StrID = null;
//Create a message showing the names and IDs of all selected files and folders
string message = null;
message = "You have selected the following files and folders: " + Constants.vbLf;
while (index <= last)
{
if (((EdmCmdData)ppoData.GetValue(index)).mlObjectID1 == 0)
{
message = message + "Folder: (ID=";
StrID = ((EdmCmdData)ppoData.GetValue(index)).mlObjectID2.ToString();
message = message + StrID + ") ";
}
else
{
message = message + "File: (ID=";
StrID = ((EdmCmdData)ppoData.GetValue(index)).mlObjectID1.ToString();
message = message + StrID + ") ";
}
message = message + ((EdmCmdData)ppoData.GetValue(index)).mbsStrData1 + Constants.vbLf;
index = index + 1;
}
//Display the message
EdmVault5 v = default(EdmVault5);
v = (EdmVault5)poCmd.mpoVault;
v.MsgBox(poCmd.mlParentWnd, message, EdmMBoxType.EdmMbt_OKOnly, CommandName);
}
}
-
Click Build > Build
Solution to build the add-in.
- Install
the add-in through the SOLIDWORKS Enterprise PDM
Administration tool:
- Open the SOLIDWORKS
Enterprise PDM Administration tool.
- Expand the vault where
you want to install this add-in and log in as Admin.
- Right-click Add-ins and click New
Add-in.
- Browse to
project_path\project_name\project_name\bin\Debug,
click project_name.dll and Interop.EdmLib.dll.
- Click Open.
- Click OK.
- Click OK.
-
Right-click inside a vault view in
Windows Explorer. First command appears in the context-sensitive
menu.
-
Right-click a file in
the vault view and click Second
command. The add-in displays a dialog similar to the following:
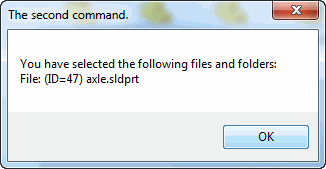
Complete
Source Code
Class1.cs
using System;
using System.Collections.Generic;
using System.Text;
using EdmLib;
using Microsoft.VisualBasic;
namespace CreateMenuCommand_CSharp
{
public class Class1 : IEdmAddIn5
{
#region IEdmAddIn5 Members
public void GetAddInInfo(ref EdmAddInInfo poInfo, IEdmVault5 poVault, IEdmCmdMgr5 poCmdMgr)
{
poInfo.mbsAddInName = "Menu command sample";
poInfo.mbsCompany = "SOLIDWORKS Corporation";
poInfo.mbsDescription = "Adds menu command items";
poInfo.mlAddInVersion = 1;
poInfo.mlRequiredVersionMajor = 5;
poInfo.mlRequiredVersionMinor = 2;
poCmdMgr.AddCmd(1000, "First command", (int)EdmMenuFlags.EdmMenu_Nothing, "This is the first command", "First command", 0, 99);
poCmdMgr.AddCmd(1001, "Second command", (int)EdmMenuFlags.EdmMenu_MustHaveSelection, "This is the second command", "Second command", 1, 99);
}
public void OnCmd(ref EdmCmd poCmd, ref Array ppoData)
{
{
string CommandName = null;
if (poCmd.mlCmdID == 1000)
{
CommandName = "The first command.";
}
else
{
CommandName = "The second command.";
}
int index = 0;
int last = 0;
index = Information.LBound(ppoData);
last = Information.UBound(ppoData);
string StrID = null;
string message = null;
message = "You have selected the following files and folders: " + Constants.vbLf;
while (index <= last)
{
if (((EdmCmdData)ppoData.GetValue(index)).mlObjectID1 == 0)
{
message = message + "Folder: (ID=";
StrID = ((EdmCmdData)ppoData.GetValue(index)).mlObjectID2.ToString();
message = message + StrID + ") ";
}
else
{
message = message + "File: (ID=";
StrID = ((EdmCmdData)ppoData.GetValue(index)).mlObjectID1.ToString();
message = message + StrID + ") ";
}
message = message + ((EdmCmdData)ppoData.GetValue(index)).mbsStrData1 + Constants.vbLf;
index = index + 1;
}
EdmVault5 v = default(EdmVault5);
v = (EdmVault5)poCmd.mpoVault;
v.MsgBox(poCmd.mlParentWnd, message, EdmMBoxType.EdmMbt_OKOnly, CommandName);
}
}
#endregion
}
}